if you are interested to learn about the react paginate
useState() hook in React is a hook that allows us to state variables in our functional components. React has two types of components. The first one is class components which are ES6 classes that extend from the React, and the second one is functional components. Class-to-components will have state and lifecycle methods: the message class extends React. use State Hook is a special function which takes the initial state as the arguments and returns it as an input array.
Syntax: The first element is the initial state, and the second one is a function used to update the state.
<strong>const</strong> [state, setState] = useState(initialState)
We pass a function as an argument if the initial state is computed. And the return value of the function is used as the initial state.
<strong>const</strong> [sum, setsum] = useState(function generateRandomInteger(){5=8+7);})
The above function is the line function which calculates the sum of numbers and it set as the initial state of it.
To use useState, we need to import useState from the react as given below:
<strong>import</strong> React, { useState } from "react"
useState() is a built-in function which comes by the React library.
<strong>import</strong> { useState } from "react";
useState() is only used inside a functional component.
Application constant = () => { const [counter, setCounter] = useState(0); // ... JSX elements };
useState() cannot work on class component. useState() returns a tuple. The parameter of the array is the current state of value. The second parameter is the function that allows updating the value of the state.
setCounter(counter + 1); // Incrementing the state value by 1
React useState() example
Application constant = () => { const [counter, setCounter] = React.useState(0); const handleClick = () => setCounter(counter + 1); return ( <> <h1>Counter: {counter}</h1> <button onClick={handleClick}>Increase</button> </> ); };
Creating the React app:
Step 1: Create a React app by using the command below:
npx create-react-app (folder name)
Step 2 After creating the project, i.e., folder name, access it by the command or syntax below:
cd folder name
The implementation of the use State () function
Application.js:
import React, { useState } of "react"; function app (props) { const [count, setRandomCount] = useState(function generate RandomInteger() { return Math.floor(Math.random() * 200); }); function clickHandler(e) { setRandomCount(Math.floor(Math.random() * 200)); } return ( <div style={{margin: 'auto', width: 100, screen: 'lock'}}> <h1> {account} </h1> <p> <button onClick={clickHandler}> Click </button> </p> </div> ); }; export default App;
Step to run the application: Run the application by using the command from the root directory of the project:
npm start
A random number initializes the first count variable to use a random function and set Random Count to update the count state. Whenever we click the onClick button, it calls the click Handler function, which resets the count variable by a random number. React’s UseState Hook allows tracking the state in a function component.
State refers to data that needs to be tracked in the application.
import useState
We first import it into our component to use use State Hook.
import { useState } from "react";
Initialize useState
We initialize our state by calling the use State on the function component. useState accepts the initial state and returns the two values which are given below:
- The current status of useCase.
- And a function that updates the state.
Example 1:
Initialize the state at the top of the function component.
import { useState } from "react"; function Favorite Color() { const [color, setColor] = useState(""); };
Notice that we are destructuring the return values of useState.
- The 1st value is color, which is the current state.
- The second value is the setColor, which is used to update the useState.
- These names of variables can have the name we like.
- We set a initial state to the empty string: useState(“).
Now we can include state anywhere in the component.
Example 2:
Use the state variable in rendered component.
import { useState } from "react"; import ReactDOM from "react-dom/client"; function Favorite Color() { const [color, setColor] = useState("red"); back <h1>My favourite color is {color}!</h1> } const root = ReactDOM.createRoot(document.getElementById('root')); root.render(<FavoriteColor /> );
Output:
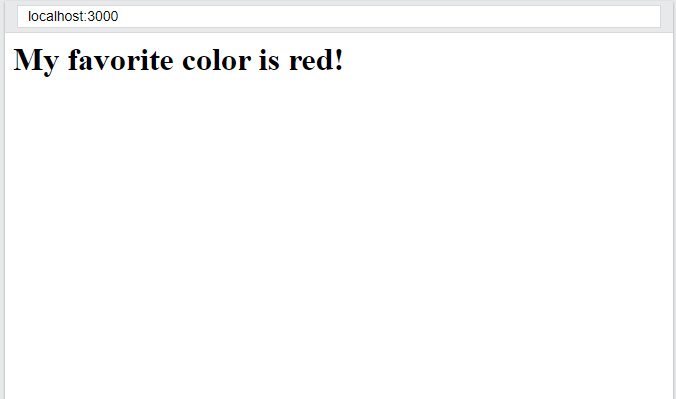
Update Status
We use our updated status feature to update status.
We can never directly update the state. E.g., color = “red” is not allowed here.
Example 3:
Use a button to update the state: import { useState } from "react"; import ReactDOM from "react-dom/client"; function Favorite Color() { const [color, setColor] = useState("red"); return ( <> <h1>My favorite color is {color}!</h1> <button type = "button" onClick={() => setColor("blue")} >Blue</button> </> ) } const root = ReactDOM.createRoot(document.getElementById('root')); root.render(<Favorite Color />);
Output:
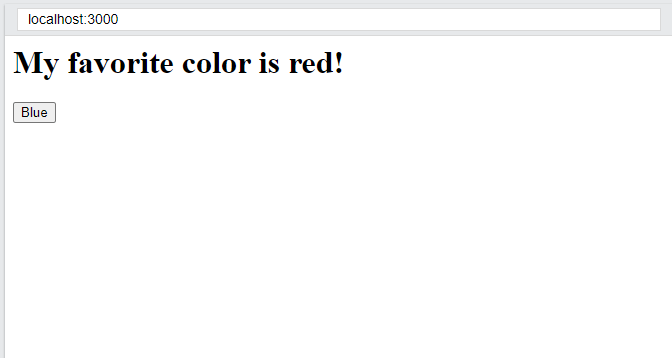
After clicking on the blue button, it turns blue like the below:
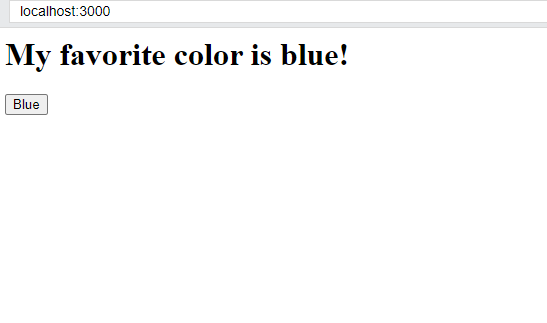
What Can State Hold?
UseState Hook is used to keep track of Strings, Like Numbers, Booleans, Arrays, Objects, and other combinations of these.
We create multi-state Hooks to track individual values.
Example 4:
Create multi-state hooks: import { useState } from "react"; import ReactDOM from "react-dom/client"; function Car() { const [brand, setBrand] = useState("Ford"); const [model, setModel] = useState("Mustang"); const [year, setYear] = useState("1964"); const [color, setColor] = useState("red"); return ( <> <h1>My {brand</h1> <p> It is a {color} {model} of {year}. </p> </> ) } const root = ReactDOM.createRoot(document.getElementById('root')); root.render(<Car />);
Output:
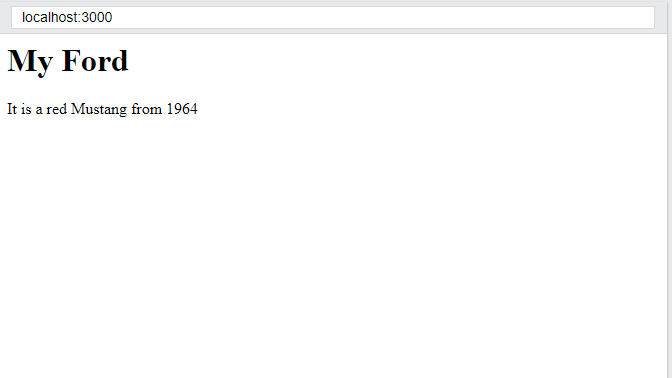
You can use a state and include an object instead!
Example 5:
Create a single Hook that will holds an object:
import { useState } from "react"; import ReactDOM from "react-dom/client"; function Car() { const [car, setCar] = useStatus({ Brand: "Ford", model: Mustang, year: "1964", Red color" }); return ( <> <h1>My {car.brand</h1> <p> It is a {car.color} {car.model} of {car.year}. </p> </> ) } const root = ReactDOM.createRoot(document.getElementById('root')); root.render(<Car />);
Output:
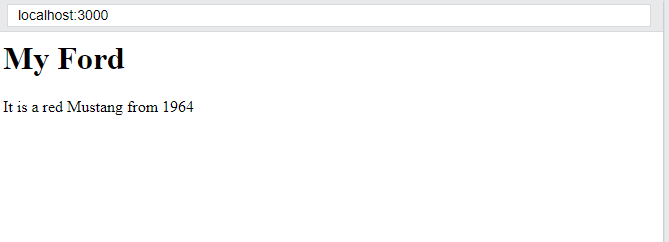
Since we are tracking a single object, we need to reference the object and the object’s property rendering the component. When the state is updated, then the entire state is overwritten.
What if we update the color of the car?
If we called setCar({color: “blue”}),
it removes the model and year from the state.
We use the JavaScript spread operator for this.
Example 6:
Here, we are using the JavaScript spread operator to update the color of the car:
import { useState } from "react"; import ReactDOM from "react-dom/client"; function Car() { const [car, setCar] = useStat U.S({ Brand: "Ford", model: mustang, year: "1964", Red color" }); const updateColor = () => { setCar(prevState => { return {...previous state, color: "blue"} }); } return ( <> <h1>My {car.brand</h1> <p> It is a {car.color} {car.model} of {car.year}. </p> <button type = "button" onClick={updateColor} >Blue</button> </> ) } const root = ReactDOM.createRoot(document.getElementById('root')); root.render(<Car />);
Output:
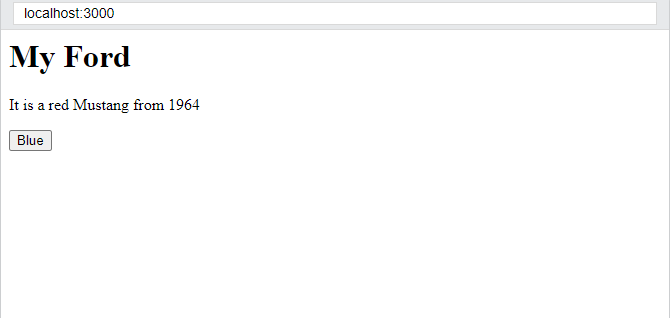
Here, we need the current value of the state, where we pass a function to the setCar function. The function receives the previous value.
We return an object, extending the state and overwriting only the car’s color.
React provides many hooks that allow to addition functionality of the components. These hooks are JavaScript functions that can import from the React package. However, hooks are available for function-based components, so they cannot be used inside a class component.
What is useState, and when do we use it?
React provides many hooks that we can use in our application. useState and useEffect are the two important hooks that we use a lot.
Use State is a function that takes only one argument at a time, the initial state, and it returns two values: the current state and a state case. If we print the useState() function in the React dev tools (console.log(useState)), we notice that it returns an array containing the argument that you have put in the useState function.
UseState Hook is used when you want to change the text after clicking a button, like creating a counter.
Simple examples of useState
You first must import it from the React package to use the useState Hooks.
Here is an example:
<strong>import</strong> React, {useState} of 'react'
Now, you can start using the Hook in the code without any problem. See the following example:
import React, { useState } from 'react' function component () { const [name, setName] = useState('Mehdi') }
Note that we are using here the unstructured ES6 array in the component. So, the variable’s name inside the array refers to the argument of the function useState. On the other hand, the setName variable refers to the function you add to update the state. We have a state name and call the setName() function.
Let’s use it by the return statement:
import React, { useState } from 'react' function component () { const [name, setName] = useState('Brad') return <h1> My name is {name} </h1> } //Returns: My name is Brad
Since function components do not have a setState() function, you must use the setName() function to update it. It is how he changes the name from “Brad” to “John”:
import React, { useState } from 'react' function component () { const [name, setName] = useState('Brad') if(name === "Brad"){ setName("John") } return <h1> My name is {name} </h1> } //Returns: My name is John
Multiple-use state
You can call useState Hook as much as you need when you have many states.
Example:
import React, {useState} of 'react' function component () { const [name, setName] = useState('Alex') const [age, setAge] = usageStatus(15) const [friends, setFriends] = useStatus(["Brad", "Mehdi"]) return <h1> My name is {name} and I am {age} </h1> //My name is Alex and I am 15 years old }
The hook receives all javaScript boolean, data types, such as string, number array, and object.
useState() concept const [counter, setCounter] = React.useState(0);
Here we will cover the basics of React.useState().
What is UseState Hook?
In React, functional components are stateless as we cannot manage their state. If we write a component when we need to add some state to it, you can use use State Hook with React 16.8. use State allows to add of the React state of the running components.
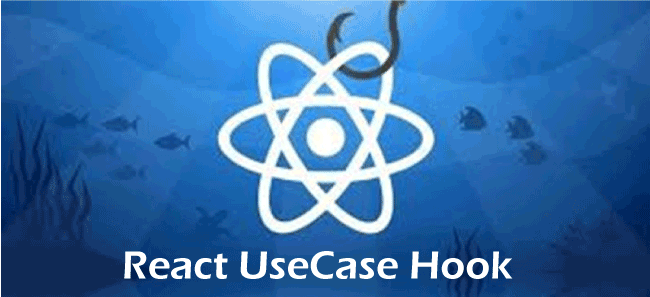
<strong>const</strong> [count, setCount] = useStatus()
- count: It is used as the current state
- setCount: It is a function that updates the state
It’s similar to this.state.count and this.setState in a class, except we see them in a pair. The useState() Hook is the initial state of Hook. The state does not have to be an object.
<strong>const</strong> [count, countSet] = useStatus(0)
Below is the code snippet for a counter that uses a class component.
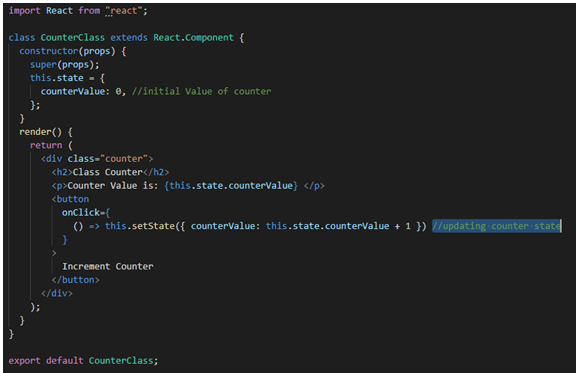
We want to implement a similar counter into the functional component.
Import the useState Hook from the React App
Declare a new state variable by calling the use State Hook. Returns a value pair, counter Value, with the number of clicks and set Counter, that updates the counter value. Initialize counter Value to zero by passing zero as the use State argument.
When the user clicks on setCounter with a new value, react will re-render the component and pass in the new value of the counterValue.
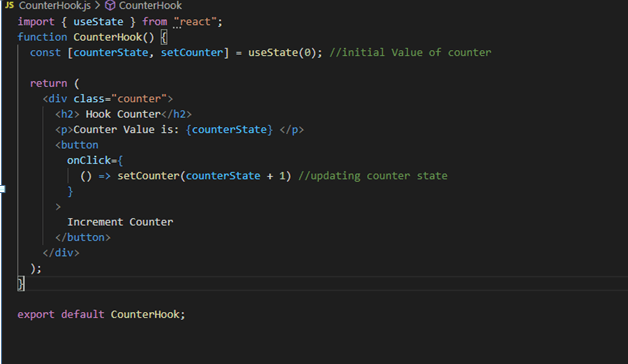
Conclusion
The use State Hook is one of the necessary and useful hooks we should know about. Also, it allows function components to do their internal state and features.