If You are interested to learn about the Scala File I/O
Inheritance is an object oriented concept which is used to reusability of code. You can achieve inheritance by using extends keyword. To achieve inheritance a class must extend to other class. A class which is extended called super or parent class. a class which extends class is called derived or base class.Syntax
class SubClassName extends SuperClassName(){ /* Write your code * methods and fields etc. */ }
Understand the Simple Example of Inheritance
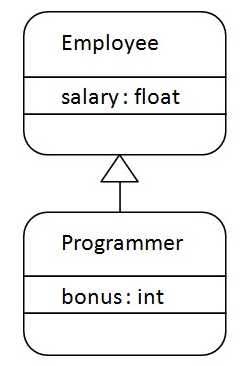
Scala Single Inheritance Example
class Employee{ var salary:Float = 10000 } class Programmer extends Employee{ var bonus:Int = 5000 println("Salary = "+salary) println("Bonus = "+bonus) } object MainObject{ def main(args:Array[String]){ new Programmer() } } <a href="https://www.javatpoint.com/scala-inheritance#"></a><a href="https://www.javatpoint.com/scala-inheritance#"></a><a href="https://www.javatpoint.com/scala-inheritance#"></a>
Output:
Salary = 10000.0
Bonus = 5000
Types of Inheritance in Scala
Scala supports various types of inheritance including single, multilevel, multiple, and hybrid. You can use single, multilevel and hierarchal in your class. Multiple and hybrid can only be achieved by using traits. Here, we are representing all types of inheritance by using pictorial form.
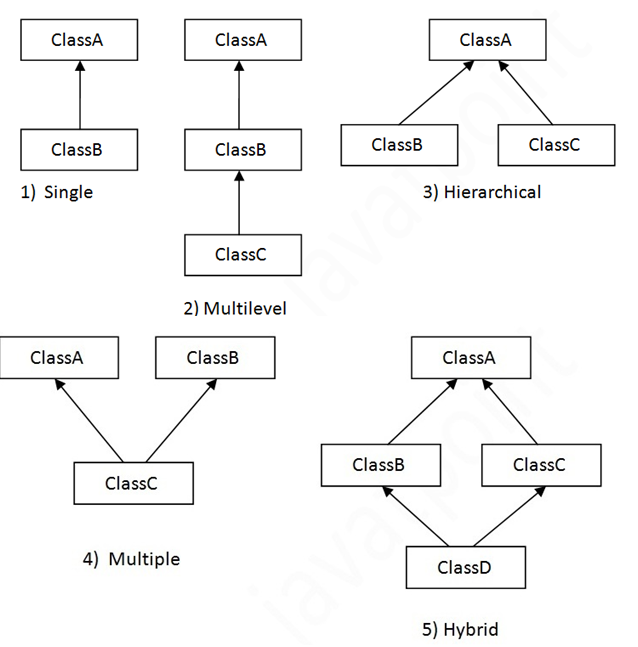
Scala Multilevel Inheritance Example
Multiple Inheritance: In Multiple inheritances, one class can have more than one superclass and acquire features from all parent classes. Scala doesn’t uphold multiple inheritances with classes, however, it can be accomplished by traits.

class A{ var salary1 = 10000 } class B extends A{ var salary2 = 20000 } class C extends B{ def show(){ println("salary1 = "+salary1) println("salary2 = "+salary2) } } object MainObject{ def main(args:Array[String]){{ var c = new C() c.show() } } <a href="https://www.javatpoint.com/scala-inheritance#"></a><a href="https://www.javatpoint.com/scala-inheritance#"></a><a href="https://www.javatpoint.com/scala-inheritance#"></a>
Output:
salary1 = 10000
salary2 = 20000
Hierarchical Inheritance
In Hierarchical Inheritance, one class fills in as a superclass (base class) for more than one subclass. When more than one classes inherit an equivalent class then this is called various Hierarchical Inheritance. For example classes B, C and D expand class A.
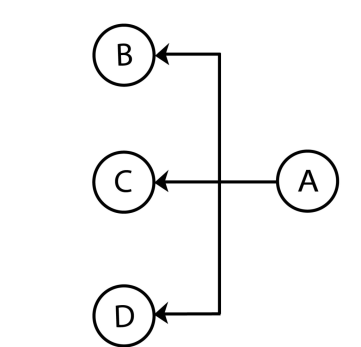
class Parent { var Name1: String = "Rahul" var Name2: String = "Shreya" } class ChildNo1 extends Parent { var Age: Int = 24 def details1() { println(" Name: " +Name1) println(" Age: " +Age) } } class ChildNo2 extends Parent { var Weight: Int = 70 def details2() { println(" Name: " +Name2); println(" Weight: " +Weight); } } object main{ def main(args: Array[String]) { val object1 = new ChildNo1() val object2 = new ChildNo2() object1.details1() object2.details2() }}
OUTPUT

Hybrid Inheritance
Hybrid Inheritance: It is a mix of at least two of the above kinds of inheritance. Since not possible with classes. In Scala, we can accomplish hybrid inheritance just through traits

class A { var numA: Int = 0; def setA(n: Int) { numA = n; } def printA() { printf("numA: %d\n", numA); } } class B extends A { var numB: Int = 0; def setB(n: Int) { numB = n; } def printB() { printf("numB: %d\n", numB); } } class C extends B { var numC: Int = 0; def setC(n: Int) { numC = n; } def printC() { printf("numC: %d\n", numC); } } class D extends A { var numD: Int = 0; def setD(n: Int) { numD = n; } def printD() { printf("numD: %d\n", numD); } } object Main { def main(args: Array[String]) { var obj1 = new C(); var obj2 = new D(); obj1.setA(10); obj1.setB(20); obj1.setC(30); obj1.printA(); obj1.printB(); obj1.printC(); obj2.setA(40); obj2.setD(50); obj2.printA(); obj2.printD(); } }
OUTPUT
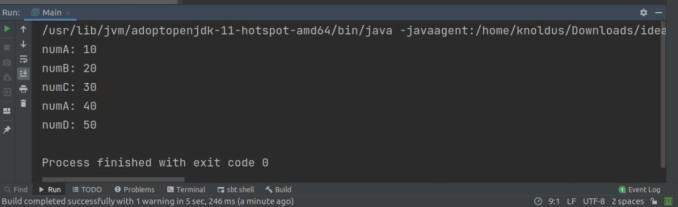