If you are interested to learn about the Replication in MongoDB
MongoDB Relationships are the representation of how the multiple documents are logically connected to each other in MongoDB. Relationships in MongoDB represent how various documents are logically related to each other. Relationships can be modeled via Embedded and Referenced approaches. Such relationships can be either 1:1, 1:N, N:1 or N:N. Let us consider the case of storing addresses for users. So, one user can have multiple addresses making this a 1:N relationship.
Following is the sample document structure of user document −
{ "_id":ObjectId("52ffc33cd85242f436000001"), "name": "Tom Hanks", "contact": "987654321", "dob": "01-01-1991" }
Following is the sample document structure of address document −
{ "_id":ObjectId("52ffc4a5d85242602e000000"), "building": "22 A, Indiana Apt", "pincode": 123456, "city": "Los Angeles", "state": "California" }
Modeling Embedded Relationships
In the embedded approach, we will embed the address document inside the user document.
> db.users.insert({ { "_id":ObjectId("52ffc33cd85242f436000001"), "contact": "987654321", "dob": "01-01-1991", "name": "Tom Benzamin", "address": [ { "building": "22 A, Indiana Apt", "pincode": 123456, "city": "Los Angeles", "state": "California" }, { "building": "170 A, Acropolis Apt", "pincode": 456789, "city": "Chicago", "state": "Illinois" } ] } })
This approach maintains all the related data in a single document, which makes it easy to retrieve and maintain. The whole document can be retrieved in a single query such as −
>db.users.findOne({"name":"Tom Benzamin"},{"address":1})
Note that in the above query, db and users are the database and collection respectively.
The drawback is that if the embedded document keeps on growing too much in size, it can impact the read/write performance.
Modeling Referenced Relationships
This is the approach of designing normalized relationship. In this approach, both the user and address documents will be maintained separately but the user document will contain a field that will reference the address document’s id field.
{ "_id":ObjectId("52ffc33cd85242f436000001"), "contact": "987654321", "dob": "01-01-1991", "name": "Tom Benzamin", "address_ids": [ ObjectId("52ffc4a5d85242602e000000"), ObjectId("52ffc4a5d85242602e000001") ] }
As shown above, the user document contains the array field address_ids which contains ObjectIds of corresponding addresses. Using these ObjectIds, we can query the address documents and get address details from there. With this approach, we will need two queries: first to fetch the address_ids fields from user document and second to fetch these addresses from address collection.
>var result = db.users.findOne({"name":"Tom Benzamin"},{"address_ids":1}) >var addresses = db.address.find({"_id":{"$in":result["address_ids"]}})

Types of MongoDB Relationships
Here we have two basic types that determine the relationship, Embedded or Reference. These types are distinguished based on how they are connected.
1. Embedded Relationships
Simply said, with MongoDB, when we try to embed a BSON document with another document, we are creating embedded relationships. There are two sub relationships with embedded relationships.
The underlying relationships are listed below:
One-to-one relationships are the most straightforward of all relationships. This is a one-to-one document, with one parent and one child for the parent.
Example:
Here, we will put a document that will link to a child document and another sub-document.
Code:
db.fun.insert({"Name":"Sulaksh", "Age":25,"Add":{"City":"Pune","State":"MA"}})
The aforementioned query will add a document to the fun collection with the specified details, and the Add key will have a child document. Let’s check and get our paperwork. The results of the find query will appear like in the following screenshot:
Output:
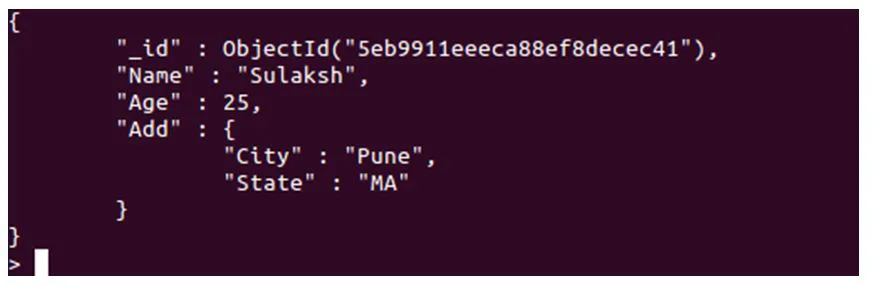
As you can see, we have a parent document which holds some data and a sub-document. The main document is the parent, and sub-document here is the child.
b. One-to-many relationships: To put it simply, one parent has several children in a One-to-Many relationship. a lot like one to one, but with plenty of child paperwork. The amount of reading operations necessary to retrieve data can be decreased by establishing a relationship using the embedded technique.
Code:
db.fun.insert(
{"Name": "Ajay",
"Age": 25,
"Add":
[
{"City": "Pune"},
{"State": "MH"}
] })
Above query will insert a new record with Name and Age, but with Add, it will insert two subdocuments. In this example, City and State will be our many relationships to the one.
Output:
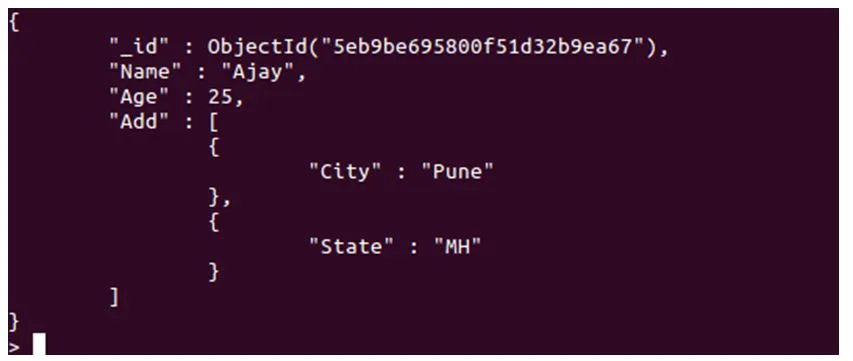
You can see that we have subdocuments that are in the add key, or One to Many relationships. An employee with various locations or phone numbers can be the simplest example of a one-to-many relationship. An employee frequently has a variety of addresses and phone numbers, and the mongodb relationship makes it simple to store this complex data in documents.
The fact that embedded relationships in MongoDB execute queries more quickly than referenced relationships is one of their main benefits. Additionally, this connection improves performance and speeds up the retrieval of results. This also holds true for sizable datasets.
2. Document Referenced Relationships
We establish two independent documents with parent and child relationships rather than placing a kid document inside a parent document. We therefore have a parent document that contains some data. We have child documents that are inserted independently in various collections but are connected by a single key from the parent document. By using this technique for building associations, you may decrease data errors and maintain data consistency.
3. Many to Many Relationships
Any two entities inside of a document can have many relations thanks to the many-to-many mongodb relationship type. Companies and clients are a simple illustration of a many-to-many relationship. While a single client may have commercial relationships with many companies, a company may have multiple clients for diverse projects and activities.
Example:
Here we will implement an example with many to many relationships. For this, we will have two separate collections with names as Authors and Books respectively.
Code:
db.authors.insert( { _id : 4, authorname : "R Tagore" } )
Above query will insert a single document, inside authors collection, which is our parent collection. We have used “_id” here, which we will use as a foreign key for child collection.
Code:
db.books.insert( {
_id : 9,
book_name : "The Post Office",
cat : [ "fiction", "play"],
artist_id : 4
})
Above query will insert a new document into our child collection, which artist_id, in connection with parent collection’s _id. Insert multiple such documents, and then we will execute an aggregation pipeline to fetch these records from two separate collections.
After inserting multiple documents, our collection will look like:
Output:
db.books.find()

4. Aggregation Pipeline
It is simply a collection of commands to be executed one by one in a sequence. We will create a pipeline to return documents from books collection, where id will be local, and artist_id will be foreign fields. Finally, we will add a $match operator, which will only return documents with an author name as R Tagore in the authors’ collections.
Code:
db.authors.aggregate( [
{ $lookup:
{ from: "books",
localField: "_id",
foreignField: "artist_id",
as: "All Books" } },
{ $match : { authorname : "R Tagore" }
}]).pretty()
Output for the above query will be the list of all the documents with the author name R Tagore in books collection.
Output:
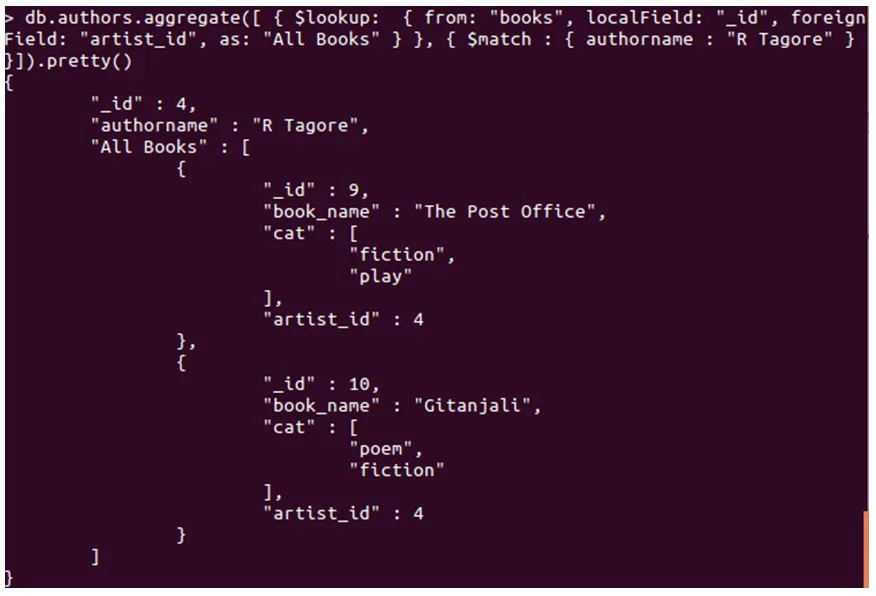
As you can see, our pipeline has returned results as expected. We have implemented many to many relationships, among books and authors.
Conclusion
MongoDB has two types of relationships. Embedded and Reference made. Every relationship has its advantages and usages. These relationships help in improving performance. Based on the situation, it implements any of the relationships as One to One, One to Many or Many to Many. Implementing this relationship has resulted in betterment with data consistency.