If you are interested to learn about the Java math
Java Conditions and If Statements
if statement is used to test the condition. It checks boolean condition: true or false. There are various types of if statement in Java. Java supports the usual logical conditions from mathematics:
- Less than: a < b
- Less than or equal to: a <= b
- Greater than: a > b
- Greater than or equal to: a >= b
- Equal to a == b
- Not Equal to: a != b
You can use these conditions to perform different actions for different decisions. Java has the following conditional statements:
- Use
if
to specify a block of code to be executed, if a specified condition is true - Use
else
to specify a block of code to be executed, if the same condition is false - Use
else if
to specify a new condition to test, if the first condition is false - Use
switch
to specify many alternative blocks of code to be executed
The if Statement
Use the if
statement to specify a block of Java code to be executed if a condition is true
.
Syntax
if (condition) { // block of code to be executed if the condition is true }
Note that if
is in lowercase letters. Uppercase letters (If or IF) will generate an error. In the example below, we test two values to find out if 20 is greater than 18. If the condition is true
, print some text:
Example
if (20 > 18) { System.out.println("20 is greater than 18"); }
We can also test variables:
Example
int x = 20; int y = 18; if (x > y) { System.out.println("x is greater than y"); }
Example explained
In the example above we use two variables, x and y, to test whether x is greater than y (using the >
operator). As x is 20, and y is 18, and we know that 20 is greater than 18, we print to the screen that “x is greater than y”.
The else Statement
Use the else
statement to specify a block of code to be executed if the condition is false
.
Syntax
if (condition) { // block of code to be executed if the condition is true } else { // block of code to be executed if the condition is false }
Example
int time = 20; if (time < 18) { System.out.println("Good day."); } else { System.out.println("Good evening."); } // Outputs "Good evening.
Example explained
In the example above, time (20) is greater than 18, so the condition is false
. Because of this, we move on to the else
condition and print to the screen “Good evening”. If the time was less than 18, the program would print “Good day”.
The else if Statement
Use the else if
statement to specify a new condition if the first condition is false
.
Syntax
if (condition1) { // block of code to be executed if condition1 is true } else if (condition2) { // block of code to be executed if the condition1 is false and condition2 is true } else { // block of code to be executed if the condition1 is false and condition2 is false }
Example
int time = 22; if (time < 10) { System.out.println("Good morning."); } else if (time < 20) { System.out.println("Good day."); } else { System.out.println("Good evening."); } // Outputs "Good evening."
Java if-else-if ladder Statement
The if-else-if ladder statement executes one condition from multiple statements.
Syntax:
if(condition1){ //code to be executed if condition1 is true }else if(condition2){ //code to be executed if condition2 is true } else if(condition3){ //code to be executed if condition3 is true } ... else{ //code to be executed if all the conditions are false }
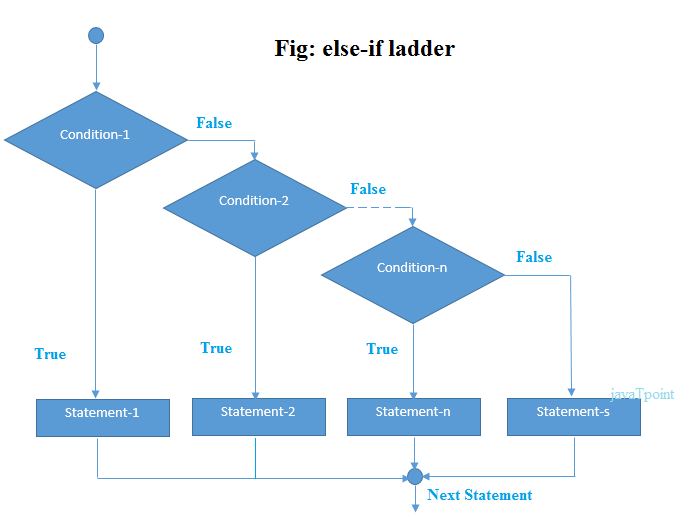
Example:
//Java Program to demonstrate the use of If else-if ladder. //It is a program of grading system for fail, D grade, C grade, B grade, A grade and A+. public class IfElseIfExample { public static void main(String[] args) { int marks=65; if(marks<50){ System.out.println("fail"); } else if(marks>=50 && marks<60){ System.out.println("D grade"); } else if(marks>=60 && marks<70){ System.out.println("C grade"); } else if(marks>=70 && marks<80){ System.out.println("B grade"); } else if(marks>=80 && marks<90){ System.out.println("A grade"); }else if(marks>=90 && marks<100){ System.out.println("A+ grade"); }else{ System.out.println("Invalid!"); } } }
Output:
C grade
Program to check POSITIVE, NEGATIVE or ZERO:
public class PositiveNegativeExample { public static void main(String[] args) { int number=-13; if(number>0){ System.out.println("POSITIVE"); }else if(number<0){ System.out.println("NEGATIVE"); }else{ System.out.println("ZERO"); } } }
Output:
NEGATIVE
Java Nested if statement
The nested if statement represents the if block within another if block. Here, the inner if block condition executes only when outer if block condition is true.
Syntax:
if(condition){ //code to be executed if(condition){ //code to be executed } }

Example:
//Java Program to demonstrate the use of Nested If Statement. public class JavaNestedIfExample { public static void main(String[] args) { //Creating two variables for age and weight int age=20; int weight=80; //applying condition on age and weight if(age>=18){ if(weight>50){ System.out.println("You are eligible to donate blood"); } } }}
Output:
You are eligible to donate blood
Example 2:
//Java Program to demonstrate the use of Nested If Statement. public class JavaNestedIfExample2 { public static void main(String[] args) { //Creating two variables for age and weight int age=25; int weight=48; //applying condition on age and weight if(age>=18){ if(weight>50){ System.out.println("You are eligible to donate blood"); } else{ System.out.println("You are not eligible to donate blood"); } } else{ System.out.println("Age must be greater than 18"); } } }
Output:
You are not eligible to donate blood
Java Short Hand If…Else (Ternary Operator)
Short Hand If…Else
There is also a short-hand if else, which is known as the ternary operator because it consists of three operands. It can be used to replace multiple lines of code with a single line, and is most often used to replace simple if else statements:
Syntax
variable = (condition) ? expressionTrue : expressionFalse;
Instead of writing:
Example
int time = 20; if (time < 18) { System.out.println("Good day."); } else { System.out.println("Good evening."); }
You can simply write:
Example
int time = 20; String result = (time < 18) ? "Good day." : "Good evening."; System.out.println(result);