If you are interested to learn about the React Props
Just like HTML DOM events, React can perform actions based on user events. React has the same events as HTML: click, change, mouseover etc. An event is an action that could be triggered as a result of the user action or system generated event. For example, a mouse click, loading of a web page, pressing a key, window resizes, and other interactions are called events.
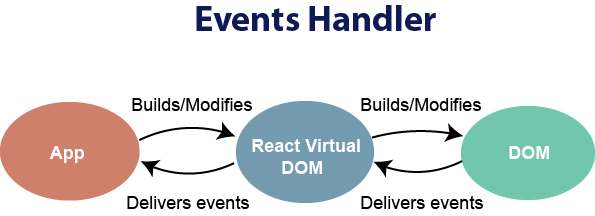
Handling events with react have some syntactic differences from handling events on DOM. These are:
- React events are named as camelCase instead of lowercase.
- With JSX, a function is passed as the event handler instead of a string. For example:
Event declaration in plain HTML:
<button onclick="showMessage()"> Hello JavaTpoint </button>
Event declaration in React:
<button onClick={showMessage}> Hello JavaTpoint </button>
3. In react, we cannot return false to prevent the default behavior. We must call preventDefault event explicitly to prevent the default behavior. For example:
In plain HTML, to prevent the default link behavior of opening a new page, we can write:
<a href="#" onclick="console.log('You had clicked a Link.'); return false"> Click_Me </a>
In React, we can write it as:
function ActionLink() { function handleClick(e) { e.preventDefault(); console.log('You had clicked a Link.'); } return ( <a href="#" onClick={handleClick}> Click_Me </a> ); }
Now let us see how to use Event in React.
Example
In the below example, we have used only one component and adding an onChange event. This event will trigger the changeText function, which returns the company name.
import React, { Component } from 'react'; class App extends React.Component { constructor(props) { super(props); this.state = { companyName: '' }; } changeText(event) { this.setState({ companyName: event.target.value }); } render() { return ( <div> <h2>Simple Event Example</h2> <label htmlFor="name">Enter company name: </label> <input type="text" id="companyName" onChange={this.changeText.bind(this)}/> <h4>You entered: { this.state.companyName }</h4> </div> ); } } export default App;
Output
When you execute the above code, you will get the following output.

After entering the name in the textbox, you will get the output as like below screen.

Adding Events
React events are written in camelCase syntax:
onClick
instead of onclick
.
React event handlers are written inside curly braces:
onClick={shoot}
instead of onClick="shoot()"
.
React:
<button onClick={shoot}>Take the Shot!</button>
HTML:
<button onclick="shoot()">Take the Shot!</button>
Example:
Put the shoot
function inside the Football
component:
function Football() { const shoot = () => { alert("Great Shot!"); } return ( <button onClick={shoot}>Take the shot!</button> ); } const root = ReactDOM.createRoot(document.getElementById('root')); root.render(<Football />);
Passing Arguments
To pass an argument to an event handler, use an arrow function.
Example:
Send “Goal!” as a parameter to the shoot
function, using arrow function:
function Football() { const shoot = (a) => { alert(a); } return ( <button onClick={() => shoot("Goal!")}>Take the shot!</button> ); } const root = ReactDOM.createRoot(document.getElementById('root')); root.render(<Football />);
React Event Object
Event handlers have access to the React event that triggered the function. In our example the event is the “click” event.
Example:
Arrow Function: Sending the event object manually:
function Football() { const shoot = (a, b) => { alert(b.type); /* 'b' represents the React event that triggered the function, in this case the 'click' event */ } return ( <button onClick={(event) => shoot("Goal!", event)}>Take the shot!</button> ); } const root = ReactDOM.createRoot(document.getElementById('root')); root.render(<Football />);