If you are interested to learn about the Installation of Cobol
COBOL uses its compiler to translate the program into an object program before the execution. To execute the COBOL program without an error, we must put the divisions in proper order. The COBOL program contains a logical structure called structure COBOL programming. It follows an organized way to do the programming. You must follow a modular programming approach, which is a subset of the procedural programming approach to do this. The COBOL program follows a hierarchical structure that is made of different components like division, section, paragraph, sentence, and characters.
All components don’t need to be present for the hierarchical relationship to exist. The division is the topmost of the component and may contain one or more sections. Similarly, a section may contain one or more paragraphs. A paragraph may contain one or more sentences, and so on
A COBOL program structure consists of divisions as shown in the following image −
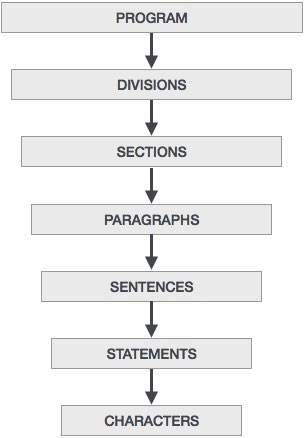
A brief introduction of these divisions is given below −
- Sections are the logical subdivision of program logic. A section is a collection of paragraphs.
- Paragraphs are the subdivision of a section or division. It is either a user-defined or a predefined name followed by a period, and consists of zero or more sentences/entries.
- Sentences are the combination of one or more statements. Sentences appear only in the Procedure division. A sentence must end with a period.
- Statements are meaningful COBOL statements that perform some processing.
- Characters are the lowest in the hierarchy and cannot be divisible.
You can co-relate the above-mentioned terms with the COBOL program in the following example −
PROCEDURE DIVISION. A0000-FIRST-PARA SECTION. FIRST-PARAGRAPH. ACCEPT WS-ID - Statement-1 -----| MOVE '10' TO WS-ID - Statement-2 |-- Sentence - 1 DISPLAY WS-ID - Statement-3 -----| .
Divisions
A COBOL program consists of four divisions.
Identification Division
It is the first and only mandatory division of every COBOL program. The programmer and the compiler use this division to identify the program. In this division, PROGRAM-ID is the only mandatory paragraph. PROGRAM-ID specifies the program name that can consist 1 to 30 characters.
IDENTIFICATION DIVISION. PROGRAM-ID. HELLO. PROCEDURE DIVISION. DISPLAY 'Welcome to future fundamentals'. STOP RUN.
Given below is the JCL to execute the above COBOL program.
//SAMPLE JOB(TESTJCL,XXXXXX),CLASS = A,MSGCLASS = C //STEP1 EXEC PGM = HELLO
When you compile and execute the above program, it produces the following result −
Welcome to future fundamentals
Environment Division
Environment division is used to specify input and output files to the program. It consists of two sections −
- Configuration section provides information about the system on which the program is written and executed. It consists of two paragraphs −
- Source computer − System used to compile the program.
- Object computer − System used to execute the program.
- Input-Output section provides information about the files to be used in the program. It consists of two paragraphs −
- File control − Provides information of external data sets used in the program.
- I-O control − Provides information of files used in the program.
ENVIRONMENT DIVISION. CONFIGURATION SECTION. SOURCE-COMPUTER. XXX-ZOS. OBJECT-COMPUTER. XXX-ZOS. INPUT-OUTPUT SECTION. FILE-CONTROL. SELECT FILEN ASSIGN TO DDNAME ORGANIZATION IS SEQUENTIAL.
Data Division
Data division is used to define the variables used in the program. It consists of four sections −
- File section is used to define the record structure of the file.
- Working-Storage section is used to declare temporary variables and file structures which are used in the program.
- Local-Storage section is similar to Working-Storage section. The only difference is that the variables will be allocated and initialized every time a program starts execution.
- Linkage section is used to describe the data names that are received from an external program.
COBOL Program
IDENTIFICATION DIVISION. PROGRAM-ID. HELLO. ENVIRONMENT DIVISION. INPUT-OUTPUT SECTION. FILE-CONTROL. SELECT FILEN ASSIGN TO INPUT. ORGANIZATION IS SEQUENTIAL. ACCESS IS SEQUENTIAL. DATA DIVISION. FILE SECTION. FD FILEN 01 NAME PIC A(25). WORKING-STORAGE SECTION. 01 WS-STUDENT PIC A(30). 01 WS-ID PIC 9(5). LOCAL-STORAGE SECTION. 01 LS-CLASS PIC 9(3). LINKAGE SECTION. 01 LS-ID PIC 9(5). PROCEDURE DIVISION. DISPLAY 'Executing COBOL program using JCL'. STOP RUN.
The JCL to execute the above COBOL program is as follows −
//SAMPLE JOB(TESTJCL,XXXXXX),CLASS = A,MSGCLASS = C //STEP1 EXEC PGM = HELLO //INPUT DD DSN = ABC.EFG.XYZ,DISP = SHR
When you compile and execute the above program, it produces the following result −
Executing COBOL program using JCL
Procedure Division
Procedure division is used to include the logic of the program. It consists of executable statements using variables defined in the data division. In this division, paragraph and section names are user-defined.
There must be at least one statement in the procedure division. The last statement to end the execution in this division is either STOP RUN which is used in the calling programs or EXIT PROGRAM which is used in the called programs.
IDENTIFICATION DIVISION. PROGRAM-ID. HELLO. DATA DIVISION. WORKING-STORAGE SECTION. 01 WS-NAME PIC A(30). 01 WS-ID PIC 9(5) VALUE 12345. PROCEDURE DIVISION. A000-FIRST-PARA. DISPLAY 'Hello World'. MOVE 'futurefundamentals' TO WS-NAME. DISPLAY "My name is : "WS-NAME. DISPLAY "My ID is : "WS-ID. STOP RUN.
JCL to execute the above COBOL program −
//SAMPLE JOB(TESTJCL,XXXXXX),CLASS = A,MSGCLASS = C //STEP1 EXEC PGM = HELLO
When you compile and execute the above program, it produces the following result −
Hello World
My name is : future fundamentals
My ID is : 12345