If you are interested to learn about the Python operators
Variables
Variables are containers for storing data values. In Python, variables are a symbolic name that is a reference or pointer to an object. The variables are used to denote objects by that name. In the above image, the variable a refers to an integer object. Suppose we assign the integer value 50 to a new variable b.
Identifier Naming
Variables are the example of identifiers. An Identifier is used to identify the literals used in the program. The rules to name an identifier are given below.
- The first character of the variable must be an alphabet or underscore ( _ ).
- All the characters except the first character may be an alphabet of lower-case(a-z), upper-case (A-Z), underscore, or digit (0-9).
- Identifier name must not contain any white-space, or special character (!, @, #, %, ^, &, *).
- Identifier name must not be similar to any keyword defined in the language.
- Identifier names are case sensitive; for example, my name, and MyName is not the same.
- Examples of valid identifiers: a123, _n, n_9, etc.
- Examples of invalid identifiers: 1a, n%4, n 9, etc.
Creating Variables
Python has no command for declaring a variable. A variable is created the moment you first assign a value to it.
Example
x = 5 y = "John" print(x) print(y)
Variables do not need to be declared with any particular type, and can even change type after they have been set.
Example
x = 4 # x is of type int x = "Sally" # x is now of type str print(x)
Casting
If you want to specify the data type of a variable, this can be done with casting.
Example
x = str(3) # x will be '3' y = int(3) # y will be 3 z = float(3) # z will be 3.0
Get the Type
You can get the data type of a variable with the type()
function.
Example
x = 5 y = "John" print(type(x)) print(type(y))
Single or Double Quotes?
String variables can be declared either by using single or double quotes:
Example
x = "John" # is the same as x = 'John'
Case-Sensitive
Variable names are case-sensitive.
Example
This will create two variables:
a = 4 A = "Sally" #A will not overwrite a
Variable Names
A variable can have a short name (like x and y) or a more descriptive name (age, carname, total_volume). Rules for Python variables:
- A variable name must start with a letter or the underscore character
- A variable name cannot start with a number
- A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ )
- Variable names are case-sensitive (age, Age and AGE are three different variables)
Example
Legal variable names:
myvar = "John" my_var = "John" _my_var = "John" myVar = "John" MYVAR = "John" myvar2 = "John"
Example
Illegal variable names:
myvar = "John" my-var = "John" my var = "John"
Assign Multiple Values
Many Values to Multiple Variables
Python allows you to assign values to multiple variables in one line:
Example
x, y, z = "Orange", "Banana", "Cherry" print(x) print(y) print(z)
One Value to Multiple Variables
And you can assign the same value to multiple variables in one line:
Example
x = y = z = "Orange" print(x) print(y) print(z)
Unpack a Collection
If you have a collection of values in a list, tuple etc. Python allows you to extract the values into variables. This is called unpacking.
Example
Unpack a list
fruits = ["apple", "banana", "cherry"] x, y, z = fruits print(x) print(y) print(z)
Output Variables
The Python print()
function is often used to output variables.
Example
x = "Python is awesome" print(x)
In the print()
function, you output multiple variables, separated by a comma:
Example
x = "Python" y = "is" z = "awesome" print(x, y, z)
Global Variables
Variables that are created outside of a function (as in all of the examples above) are known as global variables. Global variables can be used by everyone, both inside of functions and outside.
Example
Create a variable outside of a function, and use it inside the function.
x = "awesome" def myfunc(): print("Python is " + x) myfunc()
Object References
It is necessary to understand how the Python interpreter works when we declare a variable. The process of treating variables is somewhat different from many other programming languages. Python is the highly object-oriented programming language; that’s why every data item belongs to a specific type of class. Consider the following example.
- print(“John”)
Output:
John
The Python object creates an integer object and displays it to the console. In the above print statement, we have created a string object. Let’s check the type of it using the Python built-in type() function.
- type(“John”)
Output:
<class 'str'>
In Python, variables are a symbolic name that is a reference or pointer to an object. The variables are used to denote objects by that name.
Let’s understand the following example
- a = 50
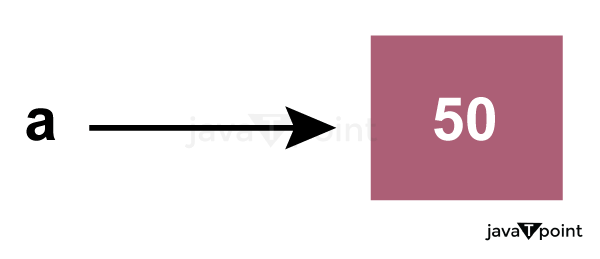
In the above image, the variable a refers to an integer object.
Suppose we assign the integer value 50 to a new variable b.Learn more
volume is gedempt
a = 50
b = a
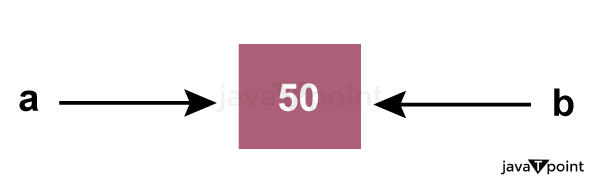
The variable b refers to the same object that a points to because Python does not create another object.
Let’s assign the new value to b. Now both variables will refer to the different objects.
a = 50
b =100
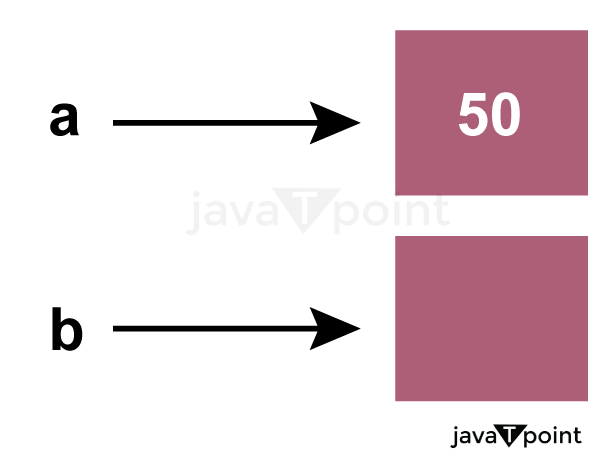
Python manages memory efficiently if we assign the same variable to two different values.https://806112e13965b13d76152749e3b4f304.safeframe.googlesyndication.com/safeframe/1-0-38/html/container.html
Object Identity
In Python, every created object identifies uniquely in Python. Python provides the guaranteed that no two objects will have the same identifier. The built-in id() function, is used to identify the object identifier. Consider the following example.
- a = 50
- b = a
- print(id(a))
- print(id(b))
- # Reassigned variable a
- a = 500
- print(id(a))
Output:
140734982691168
140734982691168
2822056960944
We assigned the b = a, a and b both point to the same object. When we checked by the id() function it returned the same number. We reassign a to 500; then it referred to the new object identifier.