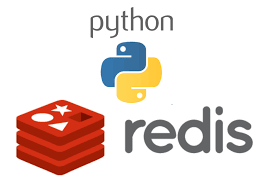
Before install python library to connect redis server, make sure you have install redis server and running successfully. There is plenty of documents are available to install redis server. Or you can follow steps here to install redis server on ubntu.
Install python library to connect redis server.
pip install redis
Create your python method or class to connect redis server.
import redis
import json
POOL = redis.ConnectionPool(host='localhost', port=6379, db=1, password=None, socket_timeout=None)
class RedisClient(object):
client = None
def __init__(self):
try:
self.client = redis.Redis(connection_pool=POOL)
self.client.client_list()
except Exception as e:
raise e
def set_key(self, key, value):
self.client.set(key, value)
def get_key(self, key):
key = self.client.get(key)
return key
Create class instance to store and get data from redis server.
# Store data to redis server
cache_storage = RedisClient()
cache_storage.set_key("phone", "1234567890")
# Get data from redis server
cache_storage.get_key("phone")
Connect redis server with python