If you are interested to learn about the React js axios
In this section, we are going to learn the use of multiple checkboxes in React. We will see the use of ReachJS to get all checked values. In our below example, we will show that how we can get value from react multiple checkboxes.
Sometimes we have to set multiple checkboxes on the basis of user requirements. We can set the options to select fruits, and users can select them according to their choices. If users want to select more than one option or multiple options from the list, they can also do this. In this case, we are required to put multiple checkboxes in ReactJS. For this, the following example will help us to understand the use of multiple checkboxes in react.
In our below example, we are going to take an array of one category, which will contain React, Laravel, PHP, Angular, etc. We will show dynamic multiple checkboxes by using the map loop. We will make a variable named “checkedItems” to store the information of the checkbox, which is selected by the user. When the form is submitted by the user, they will get the values of all selected checkboxes. The example code to do this is as follows:
Example Code:
import React, { Component } from 'react'; import { render } from 'react-dom'; class App extends Component { constructor() { super(); this.state = { categories: [ {id: 1, value: "Angular"}, {id: 2, value: "React"}, {id: 3, value: "PHP"}, {id: 4, value: "Laravel"} ], checkedItems: new Map() }; this.handleChange = this.handleChange.bind(this); this.handleSubmit = this.handleSubmit.bind(this); } handleChange(event) { var isChecked = event.target.checked; var item = event.target.value; this.setState(prevState => ({ checkedItems: prevState.checkedItems.set(item, isChecked) })); } handleSubmit(event) { console.log(this.state); event.preventDefault(); } render() { return ( <div> <h1> Examples of Multiple Checkbox in React </h1> <form onSubmit={this.handleSubmit}> { this.state.categories.map(item => ( <li> <label> <input type="checkbox" value={item.id} onChange={this.handleChange} /> {item.value} </label> </li> )) } <br/> <input type="submit" value="Submit" /> </form> </div> ); } } render(<App />, document.getElementById('root'));
When we execute the above code, we will get the following output:

How do you store multiple checkbox values in React JS?
To save multiple checkbox values in React, convert the checkbox values to a string and filter the state, and if any of the checkbox values are true, we add to an array and then change that array into the string and send that data to the server
How to Handle Multiple Checkboxes Values in React Js
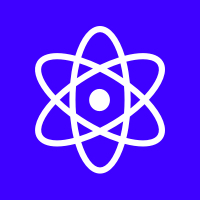
In this React Checkbox tutorial, we are going to look at how to handle and store multiple checkboxes values in React application. A checkbox is an HTML element, and It allows the user to choose one or multiple options from a limited number of choices.
We deal with various form controls to build an enterprise-level web or mobile application. There is various kind of form controls used to create an interactive form such as checkboxes, radio buttons, dropdown menus etc. A checkbox is one of the widely used HTML element in web layout, which allows us to select one or multiple values among other various options.
React Store Multiple Checkboxes Values
Let us create a basic form in React and get started with five checkboxes, and it will allow users to select multiple values using checkboxes in React app. We will also go one step further and learn to know how to store the checkboxes value in the MongoDB NoSQL database in string form.
Set up React App
Enter the following command to set up React project with CRA (create-react-app).
npx create-react-app react-multiple-checkboxes
cd react-multiple-checkboxes
Start the React app by running the following command:
npm start
After running the above command your React project will open on this URL.
Install Bootstrap 4
Install Bootstrap 4 for showing checkboxes demo in our React app
npm install bootstrap --save
Include bootstrap.min.css file in src/App.js
file:
import React, { Component } from 'react'; import '../node_modules/bootstrap/dist/css/bootstrap.min.css'; class App extends Component { render() { return ( <div className="App"> <!-- Form Goes Here --> </div> ); } } export default App;
Install Axios in React
Install Axios library in React to handle the GET, POST, UPDATE and Delete request to save the value manipulated with React checkboxes.
npm install axios --save
Setting up Checkboxes in React with Bootstrap 4
Let us create the simple form with five checkboxes elements. Keep all the below logic in src/App.js
file: In the first step we set the initial state of fruits options.
state = { isApple: false, isOrange: false, isBanana: false, isCherry: false, isAvocado: false };
React Chckbox onChange
Define React onChange
event in HTML checkbox element and set the checkbox value in it. When updated by user, this method will update the checkbox’s state.
onChangeApple = () => { this.setState(initialState => ({ isApple: !initialState.isAvocado, })); }
Go to src/App.js
file and add the following code for checkboxes demo:
import React, { Component } from 'react'; import '../node_modules/bootstrap/dist/css/bootstrap.min.css'; class App extends Component { // Checkbox Initial State state = { isApple: false, isOrange: false, isBanana: false, isCherry: false, isAvocado: false }; // React Checkboxes onChange Methods onChangeApple = () => { this.setState(initialState => ({ isApple: !initialState.isAvocado, })); } onChangeOrange = () => { this.setState(initialState => ({ isOrange: !initialState.isOrange, })); } onChangeBanana = () => { this.setState(initialState => ({ isBanana: !initialState.isBanana, })); } onChangeCherry = () => { this.setState(initialState => ({ isCherry: !initialState.isCherry, })); } onChangeAvocado = () => { this.setState(initialState => ({ isAvocado: !initialState.isAvocado, })); } // Submit onSubmit = (e) => { e.preventDefault(); console.log(this.state); } render() { return ( <div className="App"> <h2>Store Multiple Checkboxes Values in React</h2> <form onSubmit={this.onSubmit}> <div className="form-check"> <label className="form-check-label"> <input type="checkbox" checked={this.state.isApple} onChange={this.onChangeApple} className="form-check-input" /> Apple </label> </div> <div className="form-check"> <label className="form-check-label"> <input type="checkbox" checked={this.state.isAvocado} onChange={this.onChangeAvocado} className="form-check-input" /> Avocado </label> </div> <div className="form-check"> <label className="form-check-label"> <input type="checkbox" checked={this.state.isBanana} onChange={this.onChangeBanana} className="form-check-input" /> Banana </label> </div> <div className="form-check"> <label className="form-check-label"> <input type="checkbox" checked={this.state.isCherry} onChange={this.onChangeCherry} className="form-check-input" /> Cherry </label> </div> <div className="form-check"> <label className="form-check-label"> <input type="checkbox" checked={this.state.isOrange} onChange={this.onChangeOrange} className="form-check-input" /> Orange </label> </div> <div className="form-group"> <button className="btn btn-success"> Save </button> </div> </form> </div> ); } } export default App;
Set up Backend Server with Node, Express and MongoDB
We will also learn to save multiple checkboxes value in MongoDB database using Node and Express server in a React application. First set up a node server using Node, Express and MongoDB.